Access Token Authentication
API Authentication
We use standard OAuth 2.0 "Client Credentials" Flow[^1]. The API authentication is set up for
machine-to-machine connections.
Normal HUVR account credentials will not work with this flow.
Request a service account from your support contact.
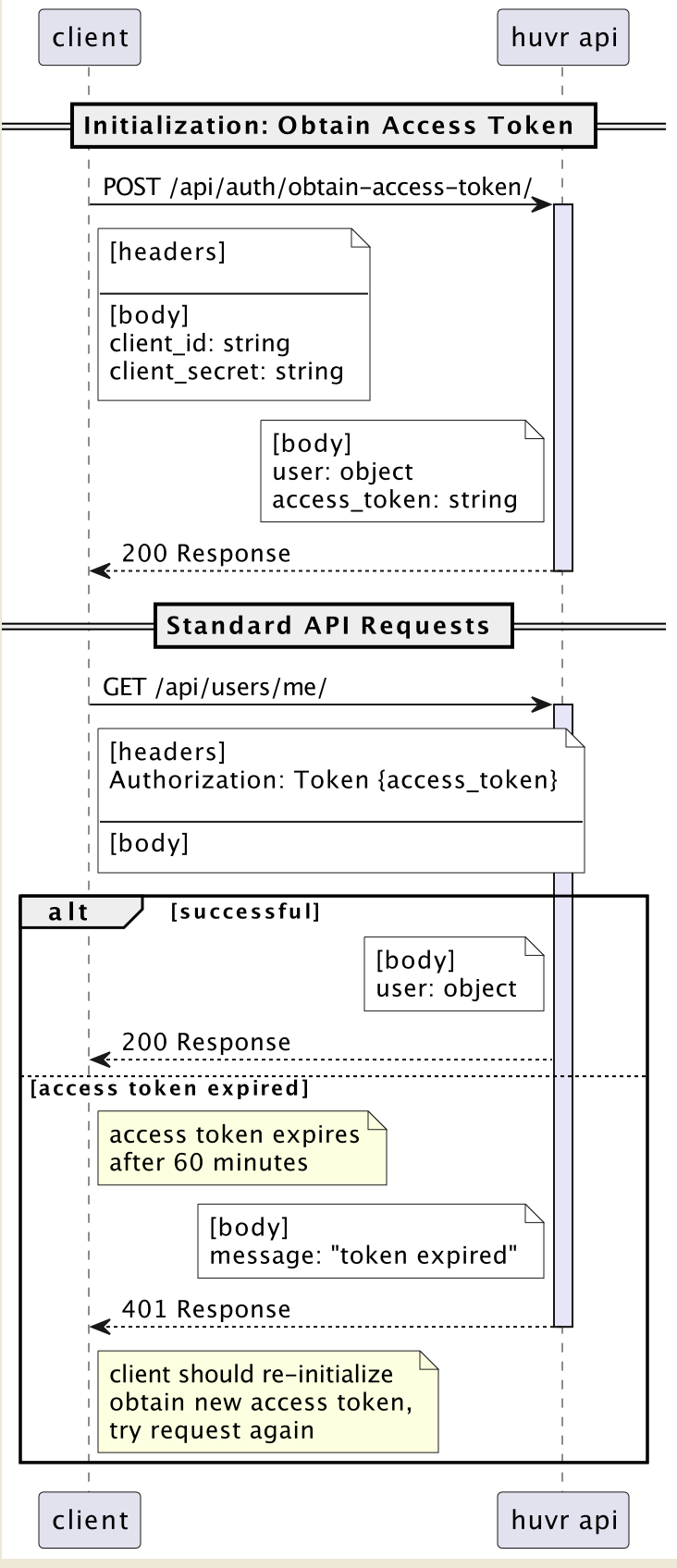
Service Account Auth Flow
0. Environment Setup
Using the following variables for the example below:
HUVR_CLIENT_ID
and HUVR_CLIENT_SECRET
will be the service accounts username and password,
HUVR_BASE_URL=https://sandbox.huvrdata.app
HUVR_CLIENT_ID=my-example-client.service-account@sandbox.huvrdata.app
HUVR_CLIENT_SECRET=<my-super-secret-key>
HUVR_BASE_URL = "https://sandbox.huvrdata.com"
HUVR_CLIENT_ID = "[email protected]"
HUVR_CLIENT_SECRET = "<my-super-secret-key>"
1. Initialize - obtain access token
Obtain a short-lived access token which will be used for all following requests.
Make a POST
request to /api/auth/obtain-access-token/
with the CLIENT_ID
/ CLIENT_SECRET
Store the returned access_token
. The access token is good for 60 minutes.
detailed endpoint reference ↗ ️
RESPONSE=$(curl --request POST \
--url "$HUVR_BASE_URL/api/auth/obtain-access-token/" \
--header 'Content-Type: application/json' \
--data "{\"client_id\": \"$HUVR_CLIENT_ID\", \"client_secret\": \"$HUVR_CLIENT_SECRET\"}")
ACCESS_TOKEN=$(echo $RESPONSE | jq --raw-output '.access_token')
import requests
response = requests.request(
method="POST",
url=f"{HUVR_BASE_URL}/api/auth/obtain-access-token/",
json={
"client_id": HUVR_CLIENT_ID,
"client_secret": HUVR_CLIENT_SECRET,
},
)
assert response.status_code == 200
access_token = response.json()["access_token"]
2. Standard request using access token for authentication
For any api-endpoint requiring authentication, add the following header to your request:
Authorization: Token <access_token>
curl --request GET \
--url "$HUVR_BASE_URL/api/users/me/" \
--header "Authorization: Token $ACCESS_TOKEN"
response = requests.request(
method="GET",
url=f"{HUVR_BASE_URL}/api/users/me/",
headers={
"Content-Type": "application/json",
"Authorization": f"Token {access_token}",
},
)
print(response.json())
Important Considerations for Access
Setting the User Agent
To avoid being blocked by security rules, it is crucial to set a descriptive User-Agent header in your requests. This helps identify your application to the server.
Example (Python using the requests library):
headers = {
'User-Agent': 'MyAPI Client/1.0' # Replace with your app's name and version
}
response = requests.get('/api/endpoint/', headers=headers)
[^1]: OAuth 2.0 - Client Credentials Grant Spec https://www.rfc-editor.org/rfc/rfc6749#section-4.4)
Updated 4 months ago